1. Hello World
Feel free to use your laptop
You are strongly encourage to work with others
When you get stuck, ask those sitting around you for help
Get used to working together in the labs
Peer teaching and peer learning has been empirically shown to be very effective
1.1. Creating a Project
Open IntelliJ
Create a new project
A start page may be shown with an option to create a new project
Or an existing project may be shown
If this is the case, select File -> New -> Project
After selecting new project, something like the following figure should be displayed
New project screen with appropriate settings selected. This figures shows the project named “cs-labs” being created and saved to the desktop, but it is recommended to save the project to a more fitting location.
After selecting “Create”, something like the following figure should be displayed
IntelliJ displaying the contents of a new project called “cs-labs”.
Add a Java class to the project
Right click src in the project explorer on left hand side of the window
Select New -> Java Class
Give the class a name, something like Lab1
By convention, always start your class names with an uppercase letter
Window to name the new class being created.
Add a
main
method to the class and have it runHello, world!
Below is an example of hello world that can be copied and pasted into the class
public static void main(String[] args) { System.out.println("Hello, world!"); }
Run the program
Select Run -> Run
One may have to select the class you want to run
Alternatively, select the green play triangle next to the main method by the line numbers
IntelliJ after the hello world program was executed. Note the
Hello, World!
output near the bottom of the window as this was the result of the program.
1.2. Practice IO
Figure out how to read standard input with a BufferedReader
Refer to the Java vs. Python topic
Write a program that will
Ask the user for two integers
Read the input
Sum the values and store the result in another variable
Print out the result
1.3. Kattis
Note
Many will have solved these already in Python. Now try to solve these problems in Java.
Don’t know how to do things in Java?
Still stumped?
Warning
In Java, the name of the class and the name of the file must be the same. Thus, for Kattis to compile the Java
submissions, the names must match. For example, the below figure shows the default file name Kattis provided as
“hello.java” (which is not following proper convention of starting all classes with an uppercase letter) and the
class name used in this lab — Lab1
. The solution here is to either rename the file by selecting “hello.java”
or rename the class.
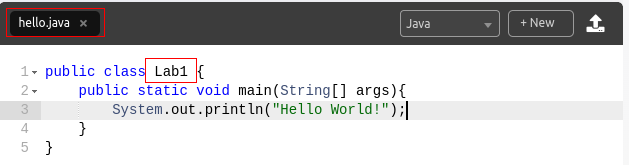
Example of the Hello World problem solution with non-matching class name and file name. If this were submitted to Kattis, it would produce a compiler error.
If not already done, create a Kattis account
Specify our school as the institution
Login to Kattis
If the above are completed, sort all the problems by difficulty and start solving.